In this laravel tutorial, we will discuss how to update and insert data by one method with an example. Here also a video explanation for laravel update or insert by the single method in Hindi.
Laravel eloquent updateOrCreate and upsert method
- updateOrCreate()
- upsert()
Laravel updateOrCreate() method
How to update or create data by one method than laravel provide updateOrCreate() method. updateOrCreate method use as when we submit some data in updateOrCreate() method then it checks this data already exists or not according to condition if already exist then it updates data otherwise it creates a new row in the table.
In updateOrCreate method first argument passes columns that are with define data exist or not and second argument column data use for create and update. If data already exists then it updates with second argument data else it create new row. Let an example of updateOrCreate() method .
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\Student; class StudentController extends Controller{ public function updateCreate(){ $id = 7; $student = Student::updateOrCreate( ['id' => $id], ['name' => 'advanced web tutorial', 'email' => 'advancedweb@gmail.com'] ); return 'Success'; } }
In above example if 7 id data exist than than it update student name and email otherwise it update same name or email.
Laravel upsert() method
Laravel upsert method is an eloquent model method and it also uses for update or creates data by single method. The difference between upsert and updateOrCreate method is upsert() method first argument takes multiple array values that are use for create and update and second argument listed columns that are uniquely identified in table and third argument pass array of columns data that are updated when matching record already available.
updateOrCreate method only use for identify one row and upsert method use when make operation one many rows. Let explain upsert method in example
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\Student; class StudentController extends Controller{ public function updateCreate(){ $student = Student::upsert( [ ['name' => 'advanced web tutorial', 'email' => 'advancedweb@gmail.com', 'age' => '15'], ['name' => 'anmol sharma', 'email' => 'anmol@gmail.com', 'age' => '30'] ] ['email'], ['name', 'email'] ); return 'Success'; } }
In above example according to second argument if same email address already available than name and email data update else data created
You read this tutorial on advanced web tutorial. here we provide laravel beginners to advanced laravel tutorial.
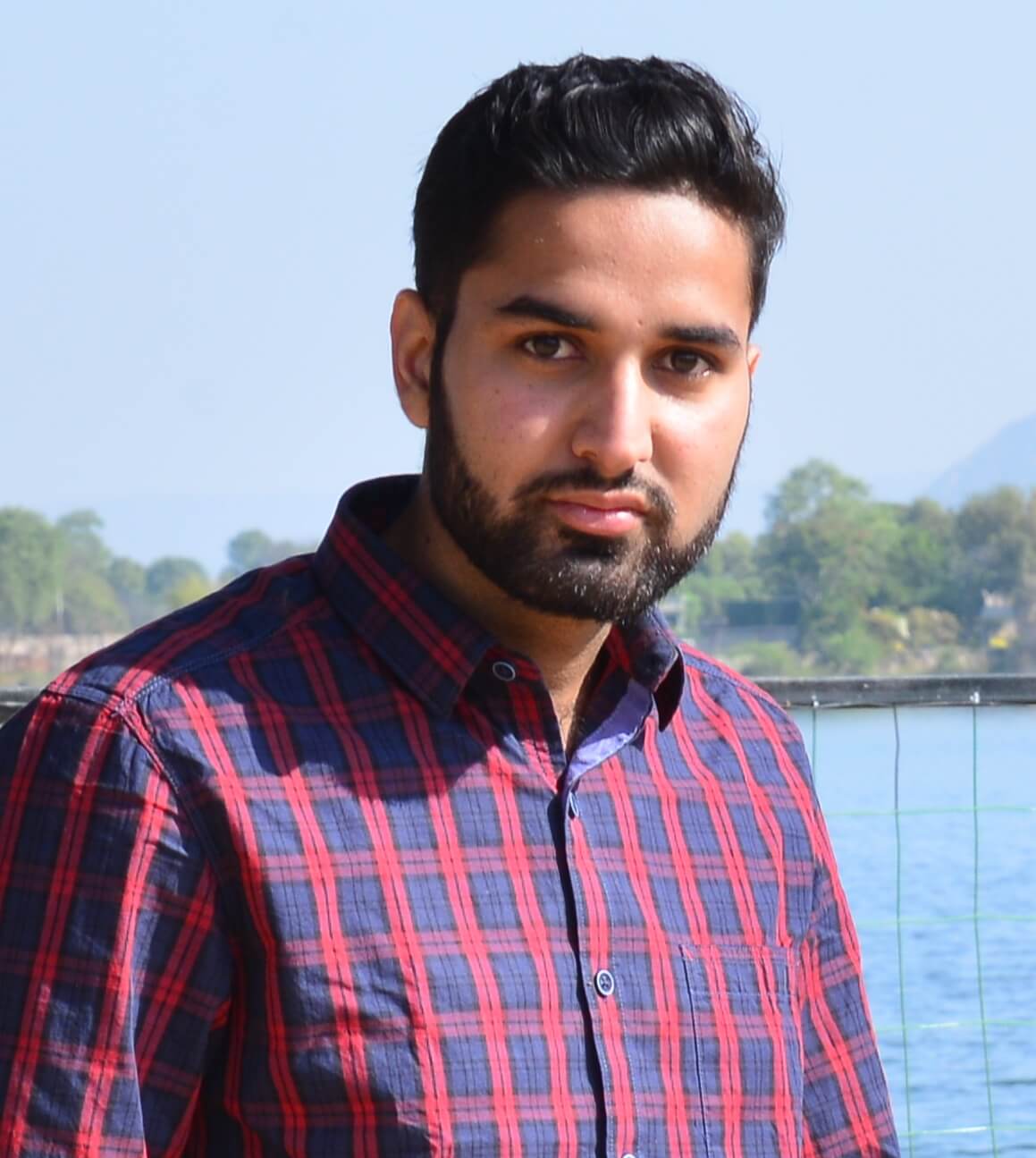
Anmol Sharma
I am a software engineer and have experience in web development technologies like php, Laravel, Codeigniter, javascript, jquery, bootstrap and I like to share my deep knowledge by these blogs.
Related tutorial links
- What is laravel eloquent model and introduction
- part 1 laravel eloquent model all configuration hindi
- part 2 laravel insert and create data by eloquent model tutorial
- part 3 laravel data retrieve method
- part 4 first, find, findOrFail and firstOrFail method
- part 5 laravel delete vs truncate method
- part 6 laravel save vs update method
- part 7 laravel updateOrCreate and upsert
- part 8 laravel chunk vs cursor method
- part 9 laravel eloquent subquery select
- part 10 laravel findOrFail vs firstOrFail
- part 11 laravel create and retrieve data
- part 12 laravel eloquent aggregate functions
- part 13 laravel isDirty vs isClean vs wasChanged method