This laravel tutorial is about eloquent model aggregate functions. We will use eloquent aggregates for database table data count, sum, max, min and average value find.
Laravel eloquent model aggregate functions
- avg()
- sum()
- min()
- max()
- count()
Laravel eloquent avg() method
Calculate the average value of a column by the laravel eloquent use avg() method. let take an example of eloquent avg aggregate function. here we use a simple avg() method on the Student model and pass column name 'marks' it gives average marks value of all students
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\Student; class StudentController extends Controller{ public function index(){ $student = Student::avg('marks'); dd($student); } }
Laravel eloquent sum() method
How to calculate the sum of all values of a column in laravel than use eloquent aggregate function sum(). more explain by an example take Employee model and calculate the sum of salary by all employees by taking salary column in eloquent sum method.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\Employee; class EmployeeController extends Controller{ public function index(){ $employee = Employee::sum('salary'); dd($employee); } }
Laravel eloquent min() method
How to calculate minimum value of an attribute than use eloquent min aggregate function. more explain by an example take Employee model and calculate minimum salary of eloloyees by min method.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\Employee; class EmployeeController extends Controller{ public function index(){ $employee = Employee::min('salary'); dd($employee); } }
Laravel eloquent max() method
Laravel eloquent max aggregate funation work same as min function. It get maximum value of given column. Let more explain by example, In example return a maximum value of salary in Employee model.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\Employee; class EmployeeController extends Controller{ public function index(){ $employee = Employee::max('salary'); dd($employee); } }
Laravel eloquent count() method
Laravel eloquent count function use for counting model data of table rows. We use simple count method with a model and it give counting result. Let an example
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\Employee; class EmployeeController extends Controller{ public function index(){ $employee = Employee::where('status','1')->count(); dd($employee); } }
also read all aggregate function in laravel query builder
You read this tutorial on advanced web tutorial. here we provide laravel beginners to advanced tutorial.
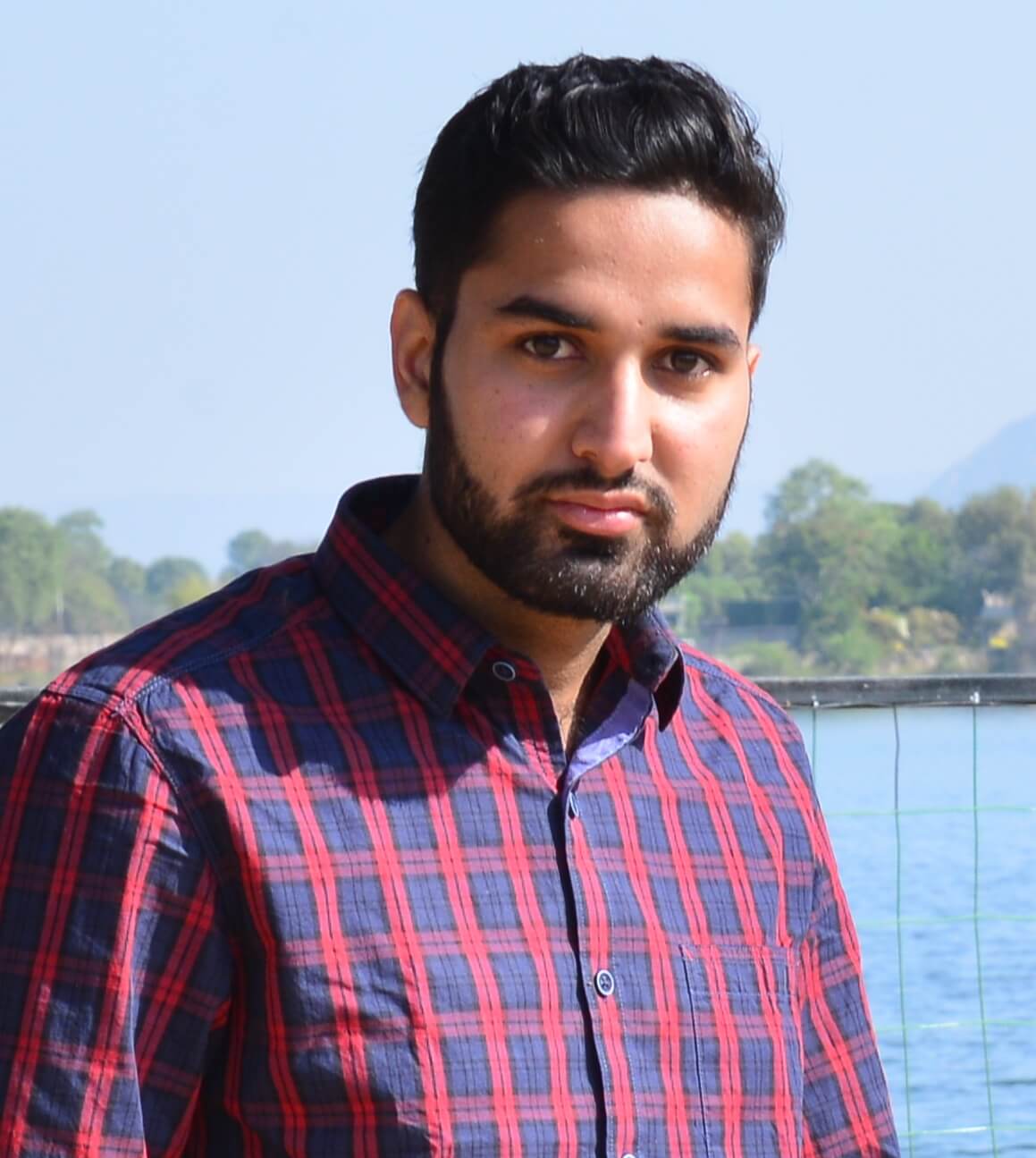
Anmol Sharma
I am a software engineer and have experience in web development technologies like php, Laravel, Codeigniter, javascript, jquery, bootstrap and I like to share my deep knowledge by these blogs.
Related tutorial links
- What is laravel eloquent model and introduction
- part 1 laravel eloquent model all configuration hindi
- part 2 laravel insert and create data by eloquent model tutorial
- part 3 laravel data retrieve method
- part 4 first, find, findOrFail and firstOrFail method
- part 5 laravel delete vs truncate method
- part 6 laravel save vs update method
- part 7 laravel updateOrCreate and upsert
- part 8 laravel chunk vs cursor method
- part 9 laravel eloquent subquery select
- part 10 laravel findOrFail vs firstOrFail
- part 11 laravel create and retrieve data
- part 12 laravel eloquent aggregate functions
- part 13 laravel isDirty vs isClean vs wasChanged method